Browser Automation with Geb, Spock & Gradle
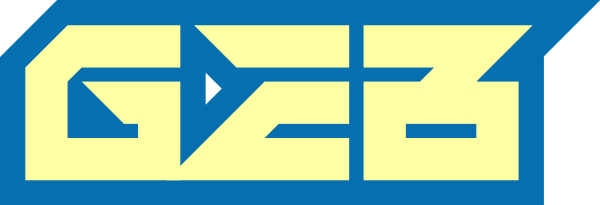
This is a repost of my blog post in 2016. Recently I was asked to do a small intro into the Geb. These are the results of a small workshop I gave at Adcubum.
So what is it?
Geb is a really nice and handy browser automation tool on top Selenium Webdriver. It provides you the full power of Selenium Webdriver. But it adds some nice features such as:
- a much more readable DSL
- Page objects to structure your test code and make it reusable.
- Integration with the Spock Framework
And how does it look like?
Page objects help you to encapsulate the content of a specific page and reuse it in several test classes. In this example GoogleFrontPage
provides an easy to use identifier for example the Google search input field. It also provides you an easy way to click on the Google search button.
class GoogleFrontPage extends geb.Page {
static url = '/'
static at = {
title == 'Google'
}
static content = {
searchInputField { $("input", name: "q") }
searchButton { $("button", name: "btnG") }
searchResultsContainer { $('#sbfrm_l') }
searchResults { $('h3.r') }
firstResult { searchResults[0] }
}
}
This enables you as a developer to write much more readable test code by writing commands like
to GoogleFrontPage
which tells Geb to browse to http://www.google.com. You can then tell Geb to enter some text into search input field of the Google Search Engine by writing
searchInputField.value = 'Geb Framework'
and then start the Google search by clicking on the
button.searchButton.click()
Putting all of this together
The listing below shows you the entire Geb test case implemented as a Spock specification. Looks neat, right?
@spock.lang.Stepwise
class GoogleSpec extends geb.spock.GebReportingSpec {
void "Visit Google.com"() {
when:
to GoogleFrontPage
then:
title == 'Google'
}
void "Make sure the query field is initially empty"() {
expect: 'The search field is initially empty'
searchInputField.text() == ''
}
void "Enter a query"() {
when: 'Enter "Geb Framework" into the search field'
searchInputField.value = 'Geb Framework'
and: 'Click the search button'
searchButton.click()
and: 'wait until the search result element is visible'
waitFor { searchResultsContainer.displayed }
then:
title == 'Geb Framework - Google Search'
and:
firstResult.text() == 'Geb - Very Groovy Browser Automation'
}
}
Where can I get that stuff?
I wrote a small starter tutorial that is hosted at https://github.com/saw303/geb-starter/. Feel free to clone it and run those tests yourself.
I wanna see more!
As initially mentioned the workshop was held in german. Therefore the recordings only are available in German language.